TopDown Shooter Week 5
Intro
For this game, I wanted to make a game with custom sprites that had a moveable player that could shoot enemies that chased you and when you kill them they respawn and keep hunting you down. This game brought a lot of its own challenges as I had a major bug where I could not get the bullets to move they would just spawn and drop. I was able to go back into the code and find the issue with the code and make sure that I gave the bullets force in the player shooting script.
Bullet script
This script made it so the bullets do kill the enemy and make them respawn. To start you need to make a variable of the enemy Prefab and this is a sprite that you drag into a folder when it’s in the object list and it gets a blue background and now you can edit its properties for all that will spawn and allows you to reference them in the script. I have it so that if the enemy is tagged as so when the bullet touches them it will destroy them but also respawn them within a randomized area that is calculated, this makes the game better as they no longer spawn in the same place every time.
using System.Collections;
using System.Collections.Generic;
using UnityEngine;
public class scr_bulletBehaviour : MonoBehaviour
{
public GameObject enemyRespawnPreFab;
void OnCollisionEnter2D(Collision2D collision)
{
if(collision.gameObject.tag == "Enemy")
{
Destroy(collision.gameObject);
Vector3 enemyRespawnPosition = new Vector3(Random.Range(-6, 6), 3, 0);
Instantiate(enemyRespawnPreFab, enemyRespawnPosition, Quaternion.identity);
Destroy(gameObject);
}
}
}
Enemy movement
For enemy movement, I made a variable of their speed which is changeable, referenced their rigid body component, referenced the player object and the player’s own rigid body. When the script runs the code the game grabs both of the rigid bodies and applies them and the game also locates the player and keeps that location. In void FixedUpdate I have it so that the enemy will turn to face the enemy with the Vector2 lookDie calculation. Then with the float step, the enemy moves towards the player with a speed calculation as well.
using System.Collections;
using System.Collections.Generic;
using UnityEngine;
public class scr_EnemyMovement : MonoBehaviour
{
public float moveSpeed = 1f;
public Rigidbody2D rb;
public GameObject player;
private Rigidbody2D playerRb;
// Start is called before the first frame update
void Start()
{
player = GameObject.Find("robot1_gun");
rb = GetComponent<Rigidbody2D>();
playerRb = player.GetComponent<Rigidbody2D>();
}
void FixedUpdate()
{
Vector2 lookDir = playerRb.position - rb.position;
float angle = Mathf.Atan2(lookDir.y, lookDir.x) * Mathf.Rad2Deg;
rb.rotation = angle;
float step = moveSpeed * Time.deltaTime;
rb.position = Vector2.MoveTowards(rb.position, playerRb.position, step);
}
}
Player movement
This movement code has the same variables as the others, one for speed, one for their rigid body, and one for the camera for making them face the curser. In void, I have the code grab the horizontal and vertical direction of my character. Then in void Fixedpdate I have to so my player can move with a calculation of speed and angles of the player to allow movement. After I dragged this onto my player I played about with the speed until I was happy with how they moved and changed the player sprite to my own drawing.
using System.Collections;
using System.Collections.Generic;
using UnityEngine;
public class scr_PlayerMovement : MonoBehaviour
{
public float moveSpeed = 5f;
public Rigidbody2D rb;
public Camera cam;
Vector2 movement;
Vector2 mousePos;
// Update is called once per frame
void Update()
{
movement.x = Input.GetAxisRaw("Horizontal");
movement.y = Input.GetAxisRaw("Vertical");
mousePos = cam.ScreenToWorldPoint(Input.mousePosition);
}
void FixedUpdate()
{
rb.MovePosition(rb.position + movement * moveSpeed * Time.fixedDeltaTime);
Vector2 lookDir = mousePos - rb.position;
float angle = Mathf.Atan2(lookDir.y, lookDir.x) * Mathf.Rad2Deg;
rb.rotation = angle;
}
}
Player shooting
The player shooting script starts with two public variables of the bulletprefab that I drag the bullet sprite into and the spawn location that I use the player for and edit it to be the end of the gun he has and also a bullet force that is changeable. I Then have it so that when the shoot button is pressed the bullets spawn with the prefab as the sprite from the spawn location and move in a staright motion follwing forward in the direction the player is facing.
using System.Collections;
using System.Collections.Generic;
using UnityEngine;
public class scr_PlayerShooting : MonoBehaviour
{
public Transform bulletSpawnLocation;
public GameObject bulletPrefab;
public float bulletForce = 20f;
// Update is called once per frame
void Update()
{
if (Input.GetButtonDown("Fire1"))
{
Shoot();
}
}
void Shoot()
{
GameObject bullet = Instantiate(bulletPrefab, bulletSpawnLocation.position, bulletSpawnLocation.rotation);
Rigidbody2D rb = bullet.GetComponent<Rigidbody2D>();
rb.AddForce(bulletSpawnLocation.right * bulletForce, ForceMode2D.Impulse);
}
}
Afterthoughts
After doing all of the code I change sprites for enemies and player by importing them as assets and dragging them into where it says sprite on them in the inspector. Overall I think I maybe could have done more with this game maybe adding a score counter and different enemy types.
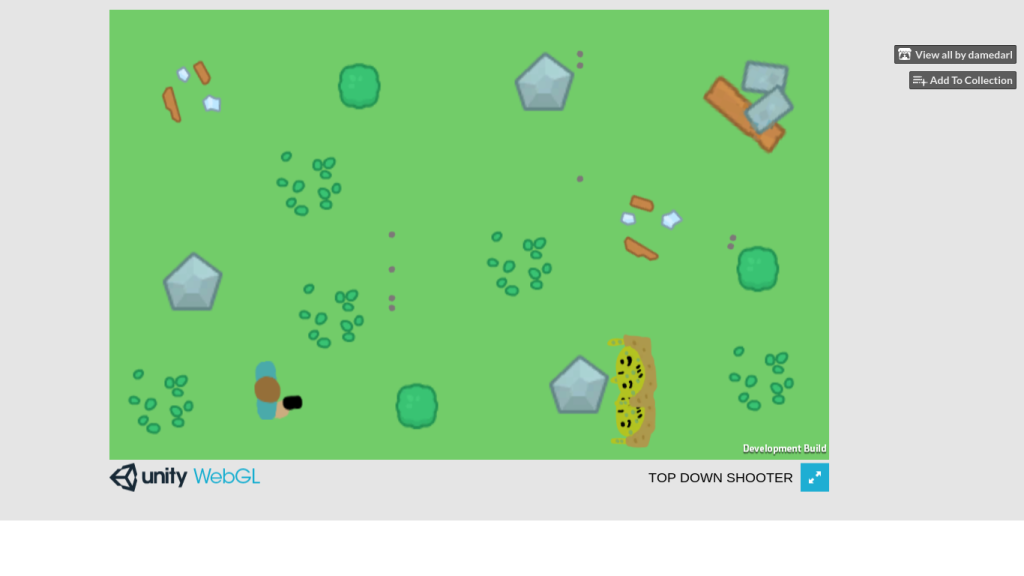
A video of gameplay.