Space Invaders Week 4
This was the game I had the most trouble making as I kept getting a lot of errors as this was my second game made in unity and I was still getting used to coding. I do believe that at the end of making this I have a better understanding of how Unity works with adding components and what different parts of the code do.
Enemy code
This is the code I used for the enemies and this gave them their basic functions of movement. This is done in the code by first adding the two variables that allow my enemies to move down and allow me to go into the inspector and edit the speed, I can edit it in the inspector since I made it public it can be edited. The transform one allows me to reference the movement later in the script. So for the movement of the enemy, I use values of -1 to +1 and then coded a calculation for the movement, this is done on the void update as every second it is running this line of code, the calculation makes it so that the position of the enemy is found and the calculation gives it its speed and allows it to move down towards the player.
using System.Collections;
using System.Collections.Generic;
using UnityEngine;
public class scr_EnemyBehaviour : MonoBehaviour
{
private Transform enemyTransform;
public float speed;
// Start is called before the first frame update
void Start()
{
enemyTransform = GetComponent<Transform>();
}
// Update is called once per frame
void Update()
{
enemyTransform.position += Vector3.down * speed;
}
}
Bullet code
This code I had a harder time understanding as I kept making silly errors that make doing the code take a lot longer than needed and this set me back overall. I learned how to fix these bugs by going back into the code and added any capitals I missed in the spellings and making sure that I used ; at the end of the line. for the start, I made a private transform for the position for later on to allow myself to code a calculation so the bullet moves and another public speed so I can change the speed of the bullet as I see fit through the course of making the game. At the start, the void start function runs and grabs the position of the bullet so when in void update the movement calculation can be done for the movement this part of the code gets the bullet moving upwards. I then coded the bullet destroying the enemy by making it so if the object is tagged as the enemy then when the bullet touches them they will disappear but then to keep the game going once destroyed I put in the values of the width of my game and made a calculation run where enemies will randomly respawn within that zone making my game feel more organic.
using System.Collections;
using System.Collections.Generic;
using UnityEngine;
public class scr_BulletBehaviour : MonoBehaviour
{
private Transform bulletTransform;
public float speed;
public GameObject enemyRespawnPrefab;
// Start is called before the first frame update
void Start()
{
bulletTransform = GetComponent<Transform>();
}
// Update is called once per frame
void FixedUpdate()
{
bulletTransform.position += Vector3.up * speed;
if (bulletTransform.position.y > 6)
{
Destroy(gameObject);
}
}
void OnTriggerEnter2D(Collider2D other)
{
if (other.tag == "Enemy")
{
Destroy(other.gameObject);
Vector3 enemyRespawnPosition = new Vector3(Random.Range(-9,9), 6, 0);
Instantiate(enemyRespawnPrefab, enemyRespawnPosition, Quaternion.identity);
Destroy(gameObject);
}
}
}
Player movement
This is for player movement and I used comments (//) to help organize the code to make it easier to fix if it was to break as I can see what does what. First is the variables, they is thr private transform for movement, public speed for inputing a speed and a private flaot moveH again for movment calucations of the character. Then the bullet creation has its own variables of a slot for me to add a bullet object, a transform of the position of the bulley, a public fire rate that I can change and a private float for next fire. In void start the code it only grabs the position of the character. In void update to get my player having controls to move them I take the moveH value and times it Input.GetAxis and this gives the movement a value that is either -1 that is left or +1 which is right. Since this makes the player too fast i multiple the mvmement by speed and make it a small decimal number to make them move alot slower. For the shooting button I use the “jump” button which is space and if it is pressed it runs the true code that will spawn the bullet at the player then the bullet script will run shooting up. I need to drag the bullet into the insepctor under bulletFired and then the player is done.
using System.Collections;
using System.Collections.Generic;
using UnityEngine;
public class NewBehaviourScript : MonoBehaviour
{
//player movment variables
private Transform playerTransform;
public float speed;
private float moveH;
//bullet creation variables
public GameObject bulletFired;
public Transform bulletSpawn;
public float fireRate;
private float nextFire;
// Start is called before the first frame update
void Start()
{
playerTransform = GetComponent<Transform>();
}
// Update is called once per frame
void Update()
{
float moveH = Input.GetAxis("Horizontal");
Vector3 movement = new Vector3(moveH, 0, 0);
playerTransform.position += movement * speed;
if (Input.GetButton("Jump") && Time.time > nextFire)
{
nextFire = Time.time + fireRate;
Instantiate(bulletFired, bulletSpawn.position, bulletSpawn.rotation);
}
}
void FixedUpdate()
{
playerTransform.position += Vector3.right * moveH * speed;
}
}
This is the code I used to make it so that when the players go past you it restarts the game killing you. To do this I added void OnTriggerEnter2D as this means when the enemy runs into the wall behind the player has this code the script trigger. My script has it so that when the “player” marked object collides with whatever his this script attached it causes the scene to restart which gives the effect of the player has died. I used a video found online to implement this and adapted it for my needs. I used another player sprite and stretched it out just under the player out of view so it looks like when the enemies get past you the game restarts giving you an objective.
using System.Collections;
using System.Collections.Generic;
using UnityEngine;
using UnityEngine.SceneManagement;
public class killPlayer : MonoBehaviour
{
public int Respawn;
// Start is called before the first frame update
void Start()
{
}
// Update is called once per frame
void Update()
{
}
void OnTriggerEnter2D(Collider2D other)
{
if(other.CompareTag("Player"))
{
SceneManager.LoadScene(Respawn);
}
}
}
The last upgrade I did to the game was adding two squares on either side of the camera and giving them a box collider making them a solid object so that my player cannot pass through them keeping them on screen as before you could just fly away forever but the boxes keep you in the screen. I drew my own art for the game to make it look cuter and make it a dog who is shooting bones at the other dogs to eat.
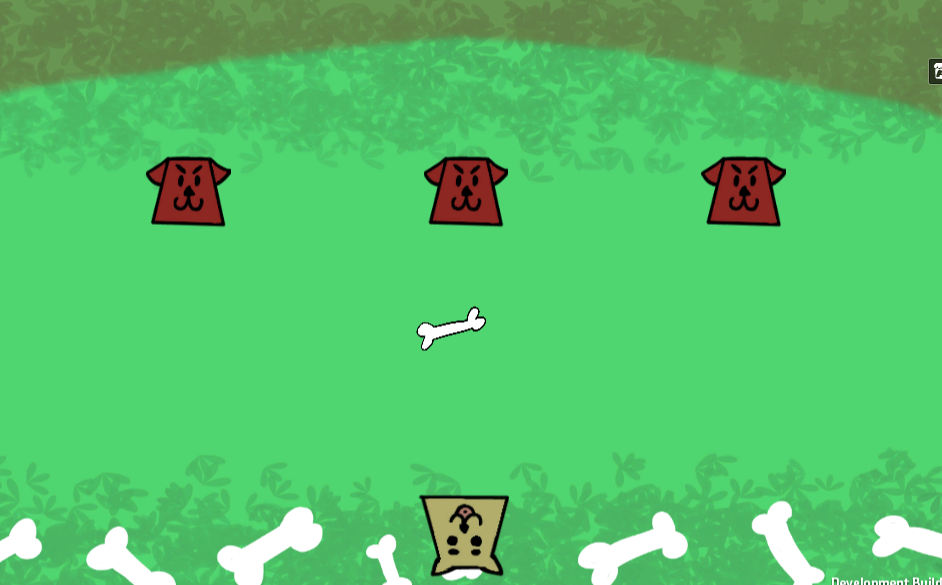
This is a video showing gameplay.