Cookie Clicker Week 3
Intro
This is the first game I have ever made so took me the longest of about 6 hours overall, my goal was to make a basic game that you can click an object and the numbers go up, and two upgrades one that automatically makes your number go up and one that makes your clicks do more. This game did break every time I tried it as I kept making very simple mistakes and learned by the end to check every line has a ; and to make sure to capitalize the write letters and not to make spelling mistakes and use spacing correctly.
This script adds clicks to the total automatically.
First the variables of if addingcats is false, the amount increased and the clicking. In update they is a function checking for if adding cats is true and if so then it increases the number by the amount clicked. This script is key for the calculation for the clicking to work and is the basic function of the gameplay of clicking makes the number go up.
using System.Collections;
using System.Collections.Generic;
using UnityEngine;
public class scr_AutoPizza : MonoBehaviour
{
public bool addingCats = false;
public int increaseAmmount = 1;
public scr_Clicking click;
// Update is called once per frame
void Update()
{
if (addingCats == false)
{
addingCats = true;
StartCoroutine(FeedCat());
}
}
IEnumerator FeedCat()
{
click.counterNumber += increaseAmmount;
yield return new WaitForSeconds(1);
addingCats = false;
}
}
Upgrade
This upgrade makes it so when it is bought the number of cats goes up automatically. First variables of two different scrips I will need to link to it, the text that shows, all of its values, name, and price. In void start it makes it so the nextcatpersec is set to one. In public void they is an if statement for if the price is the same as the cat amount you can buy the upgrade that takes the amount of cats away and makes it go up by 1 each second. Then I code the text display that pops up when you hover over the auto feeder icon and that says what the item does that changes as you buy more as it does more and more.
using System.Collections;
using System.Collections.Generic;
using UnityEngine;
using UnityEngine.UI;
public class scr_BuyUpgrade_AutoClick : MonoBehaviour
{
public scr_Clicking click;
public scr_AutoPizza auto;
public Text upgradeInfo;
public float currentPrice, powerOf, multiplier;
public string upgradeName;
public int nextCatPerSec;
private float basePrice;
// Start is called before the first frame update
void Start()
{
nextCatPerSec = 1;
basePrice = currentPrice;
}
public void PurchaseUpgrade()
{
if (click.counterNumber >= currentPrice)
{
click.counterNumber -= currentPrice;
powerOf++;
auto.increaseAmmount = nextCatPerSec;
nextCatPerSec += 1;
currentPrice = Mathf.Round(basePrice * Mathf.Pow(2.05f, powerOf));
UpdateText();
}
}
public void OnMouseEnter()
{
UpdateText();
}
public void OnMouseExit()
{
upgradeInfo.text = " ";
}
void UpdateText()
{
upgradeInfo.text = upgradeName + "\n" + "$ " + currentPrice + " \n" + "Upgrade to auto generate " + nextCatPerSec + " cats per click";
}
// Update is called once per frame
void Update()
{
}
}
Upgrade 2
This upgrade is similar to the other so I will not repeat myself by talking about the variables. The part of this upgrade that is different is that in public void I have it so when I can buy it and do it each time I click it is time by 1.5 increases my clicking power.
using System.Collections;
using System.Collections.Generic;
using UnityEngine;
using UnityEngine.UI;
public class scr_BuyUpgrade_PerClick : MonoBehaviour
{
public scr_Clicking click;
public Text upgradeInfo;
public float currentPrice, clickPower, PowerOf;
public string upgradeName;
public float basePrice;
public void Start ()
{
basePrice = currentPrice;
}
public void PurchaseUpgrade()
{
if (click.counterNumber >= currentPrice)
{
click.counterNumber -= currentPrice;
PowerOf++;
click.ammountPerClick += clickPower;
//currentPrice = Mathf.Round(currentPrice * 2f);
currentPrice = Mathf.Round(basePrice * Mathf.Pow(1.15f, PowerOf));
}
}
public void OnMouseEnter()
{
UpdateText();
}
public void OnMouseExit()
{
upgradeInfo.text = " ";
}
void UpdateText()
{
upgradeInfo.text = upgradeName + "\n" + "Upgrade to " + clickPower + " times cats per click!";
}
}
Clicking
This code allows me to click on the cat and the number goes up. First as allways I put in my variables and they are a public text, number and an amount per click that is defult set to one.In the void update it displays the number of clicks in the box along with my text chosen. Then in void public update it does the clauction that the number is the amount of times clicked for adding the clicks up.
using System.Collections;
using System.Collections.Generic;
using UnityEngine;
using UnityEngine.UI;
public class scr_Clicking : MonoBehaviour
{
public Text counter;
public float counterNumber;
public float ammountPerClick = 1.0f;
// Update is called once per frame
void Update()
{
counter.text = counterNumber + " Cats Fed";
}
public void Clicked()
{
counterNumber += ammountPerClick;
}
}
Afterthoughts
Once I got my work done I edited the sprites by changing them in the inspector to make my game look a lot cuter and have a more solid theme. I did not upgrade this game but if I was to I would like to have a goal and then a well-done scene and to add another upgrade that randomly appears and when you click on it you get a times 10 bonus for 10 seconds. For my first game though I am very proud of it as it does work and it was the first time I ever coded.
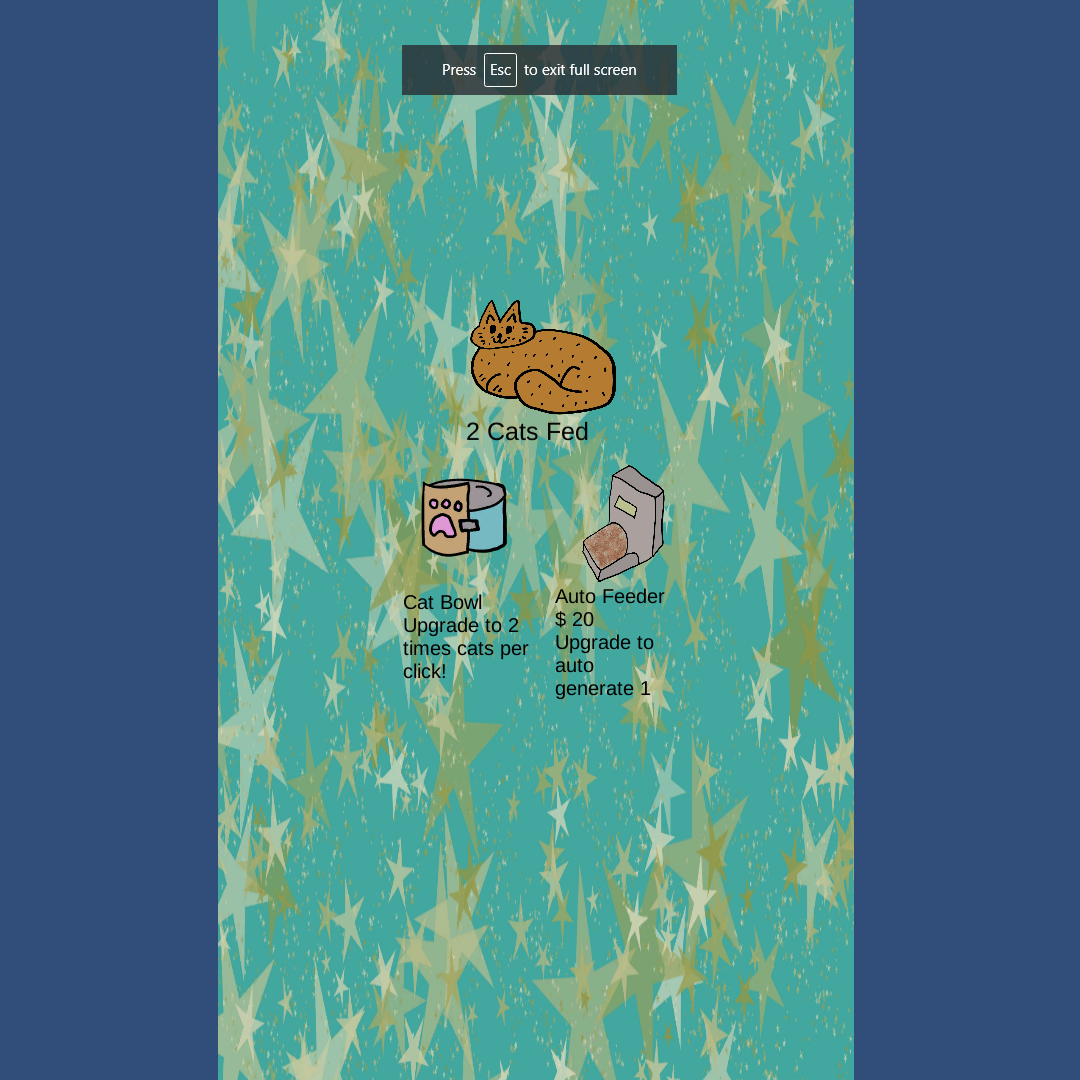